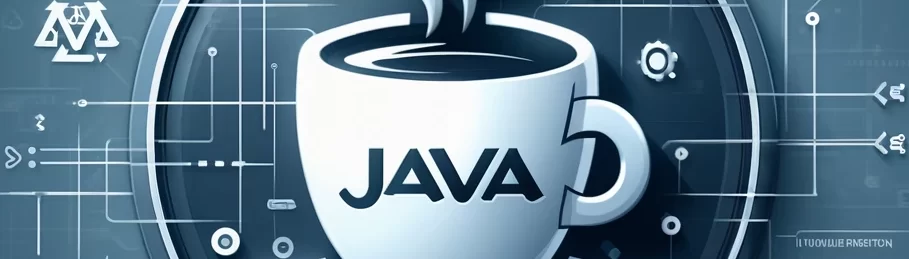
What is java? well Java is a high-level, class-based, object-oriented programming language that is designed to have as few implementation dependencies as possible. It is a general-purpose programming language that is widely used for building robust, secure, and scalable applications.
History and Evolution of Java
Java was developed by James Gosling and his team at Sun Microsystems in 1995. Initially designed for interactive television, it soon became apparent that Java was well-suited for the burgeoning web, thanks to its portability and security features.
Features of Java
Java is renowned for several key features:
- Platform Independence: Java programs are compiled into bytecode that can run on any device with a Java Virtual Machine (JVM), making it platform-independent.
- Object-Oriented: Java supports the principles of Object-Oriented Programming (OOP) such as inheritance, polymorphism, encapsulation, and abstraction.
- Robust and Secure: Java has strong memory management, exception handling, and a security framework that makes it highly reliable.
- Multithreading: Java provides built-in support for multithreaded programming, allowing concurrent execution of two or more threads.
Uses of Java
Java is used in a wide array of applications, from mobile and desktop applications to large-scale enterprise systems and scientific computing. Some common applications include:
- Web Applications: Using frameworks like Spring and Hibernate.
- Mobile Applications: Especially Android apps, built with Java or Kotlin.
- Enterprise Applications: Banking, retail, and other enterprise-level applications.
- Big Data Technologies: Hadoop and other big data platforms.
Getting Started with Java
To start coding in Java, you need to install the Java Development Kit (JDK) and an Integrated Development Environment (IDE) like IntelliJ IDEA, Eclipse, or NetBeans.
Here’s a simple “Hello, World!” example in Java to get you started:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Step-by-Step Explanation:
- public class HelloWorld: Defines a public class named
HelloWorld
. - public static void main(String[] args): The main method is the entry point of any Java application. It’s a static method that takes an array of strings as arguments.
- System.out.println(“Hello, World!”);: This line prints “Hello, World!” to the console.
To run this code, save it in a file named HelloWorld.java
, compile it using javac HelloWorld.java
, and run it with java HelloWorld
.
Frequently Asked Questions (FAQ)
1. What is Java used for?
- Java is used for web development, mobile development, enterprise applications, scientific computing, and more.
2. What are the features of Java?
- Java is platform-independent, object-oriented, robust, secure, and supports multithreading.
3. How do I get started with Java?
- Install the Java Development Kit (JDK), set up an IDE, and start writing and running Java programs.
4. Is Java the same as JavaScript?
- No, Java and JavaScript are different languages with distinct purposes. Java is a standalone programming language, while JavaScript is primarily used for web development.